Mastering Scala: Core Concepts Every Student Should Know
When diving into the world of Scala programming, students often seek guidance and support to navigate through its complex features and concepts. A scala assignment helper can be an invaluable resource for demystifying these concepts and assisting with coursework. Scala, with its blend of object-oriented and functional programming paradigms, offers a rich landscape for coding that can be both exciting and challenging. To truly excel, understanding the foundational elements of Scala is crucial. In this blog, we'll explore essential Scala concepts that every student should master to build a strong programming foundation.
Visit: https://www.programminghomewor....khelp.com/scala-assi
1. Scala's Basics: Syntax and Structure
Before delving into advanced topics, it's essential to get comfortable with Scala's basic syntax and structure. Scala combines elements of both functional and object-oriented programming, which means understanding its syntax is key to leveraging its full potential.
1.1 Variables and Data Types
In Scala, variables can be declared using val or var. val is used for immutable variables, while var is used for mutable variables. For instance:
scala
Copy code
val pi: Double = 3.14159
var counter: Int = 0
Scala supports a range of data types including Int, Double, Boolean, Char, and String. Type inference is a powerful feature in Scala, allowing the compiler to deduce types automatically:
scala
Copy code
val message = "Hello, Scala!" // Type inferred as String
1.2 Functions and Methods
Functions in Scala are defined using the def keyword. They can be parameterless or take parameters, and their return types are often inferred:
scala
Copy code
def greet(name: String): String = s"Hello, $name!"
Methods can be defined within classes or objects and can have additional features such as overloading:
scala
Copy code
class Greeter {
def greet(): String = "Hello!"
def greet(name: String): String = s"Hello, $name!"
}
2. Object-Oriented Programming in Scala
Scala’s object-oriented programming (OOP) features allow students to design scalable and maintainable code.
2.1 Classes and Objects
Scala classes are defined with the class keyword and can contain fields and methods. Here's a simple example:
scala
Copy code
class Person(val name: String, var age: Int) {
def celebrateBirthday(): Unit = {
age += 1
println(s"Happy $age birthday, $name!"
}
}
Scala's object keyword defines singleton objects. They are often used for utility functions or to implement the Singleton pattern:
scala
Copy code
object MathUtils {
def add(x: Int, y: Int): Int = x + y
}
2.2 Inheritance and Traits
Scala supports inheritance and allows classes to extend other classes. Traits are Scala's way of achieving multiple inheritance and can be mixed into classes:
scala
Copy code
trait Greeter {
def greet(): String = "Hello!"
}
class Person(name: String) extends Greeter {
override def greet(): String = s"Hello, $name!"
}
3. Functional Programming in Scala
Scala’s functional programming features offer powerful tools for handling data and improving code readability.
3.1 Immutable Collections
Immutability is a cornerstone of functional programming. Scala provides several immutable collections such as List, Set, and Map. Here's how you can work with them:
scala
Copy code
val numbers = List(1, 2, 3, 4)
val squares = numbers.map(n => n * n)
3.2 Higher-Order Functions
Higher-order functions are functions that take other functions as parameters or return functions. Scala’s functional programming capabilities are exemplified by:
scala
Copy code
def applyOperation(x: Int, operation: Int => Int): Int = operation(x)
val double = (x: Int) => x * 2
val result = applyOperation(5, double) // result is 10
3.3 Pattern Matching
Pattern matching in Scala is a powerful feature that allows for sophisticated control flow and data extraction. It is used similarly to switch statements in other languages but is much more versatile:
scala
Copy code
def describe(x: Any): String = x match {
case 1 => "one"
case "hello" => "greeting"
case _: Int => "integer"
case _ => "unknown"
}
4. Concurrency and Parallelism
Scala’s concurrency model is designed to simplify writing concurrent and parallel code, primarily through the use of actors and Futures.
4.1 Actors
Actors provide a higher-level abstraction for concurrent programming. They enable you to design systems where components communicate by passing messages:
scala
Copy code
import akka.actor._
class MyActor extends Actor {
def receive = {
case "hello" => println("Hello back!"
case _ => println("Unknown message"
}
}
val system = ActorSystem("MySystem"
val myActor = system.actorOf(Props[MyActor], "myActor"
myActor ! "hello"
4.2 Futures and Promises
Futures and Promises are used for asynchronous programming in Scala. They allow you to handle operations that may take time to complete:
scala
Copy code
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val future = Future {
// Simulate long computation
Thread.sleep(100
42
}
future.onComplete {
case Success(value) => println(s"Computation result: $value"
case Failure(exception) => println(s"Computation failed: $exception"
}
5. Advanced Scala Features
For students looking to dive deeper into Scala, several advanced features can help refine their skills and understanding.
5.1 Implicits
Implicits in Scala allow you to define parameters that are automatically provided by the compiler. They can be used for various purposes, including dependency injection and extension methods:
scala
Copy code
implicit val defaultMultiplier: Int = 2
def multiply(x: Int)(implicit multiplier: Int): Int = x * multiplier
val result = multiply(3) // Uses implicit value of 2, result is 6
Conclusion
For those struggling with Scala coursework, a scala assignment helper can provide additional support and insights to tackle complex problems and refine your understanding of Scala. Embracing these concepts not only prepares you for academic success but also equips you with skills valuable in real-world programming scenarios.
Reference: https://www.programminghomewor....khelp.com/blog/top-s
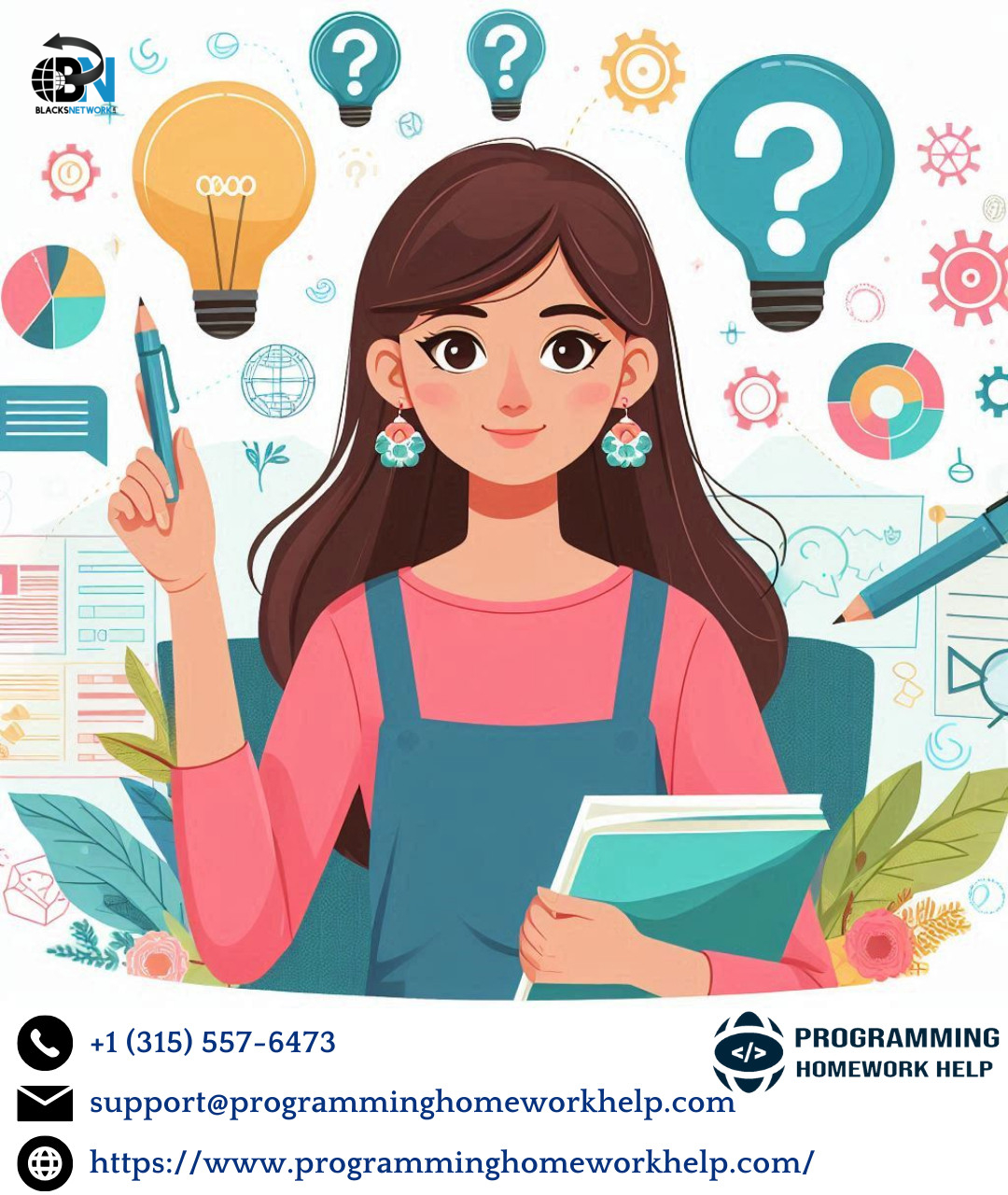