Advanced Techniques in Non-Linear Regression for Water Flow Analysis
When dealing with complex systems like water flow, linear regression often falls short due to its simplicity. Non-linear regression, however, provides a robust alternative, capturing the intricacies of such systems more effectively. For students and professionals alike, understanding this powerful analytical tool is crucial. Whether you're tackling assignments or real-world problems, online machine learning assignment help can be invaluable in mastering these concepts. Let's dive into the essentials of non-linear regression for water flow analysis, from prerequisites to practical applications.
Visit: https://www.programminghomewor....khelp.com/machine-le
Prerequisites
Before embarking on non-linear regression, a solid grasp of Python programming and fundamental statistical concepts is essential. Familiarity with libraries such as NumPy, Pandas, Matplotlib, and SciPy will significantly ease the process. Additionally, understanding the basics of regression analysis and the differences between linear and non-linear models sets a strong foundation.
Python Programming: Proficiency in Python is crucial, as it is the primary language used in this analysis. Ensure you are comfortable with Python syntax and basic programming constructs.
Statistical Knowledge: Basic statistical knowledge, particularly in regression analysis, is necessary to understand the theoretical underpinnings of non-linear regression.
Libraries: Mastery of Python libraries like NumPy for numerical computations, Pandas for data manipulation, Matplotlib for plotting, and SciPy for scientific computations is required.
Data Preparation
Data preparation is the cornerstone of any analytical process. For water flow analysis, this involves collecting relevant data, cleaning it, and ensuring it's in a format suitable for analysis.
Data Collection: Gather historical water flow data, which could include variables such as time, water level, temperature, and precipitation. Reliable sources include governmental databases, environmental monitoring agencies, and research institutions.
Data Cleaning: Clean the data to handle missing values, remove duplicates, and correct any inconsistencies. This step ensures the integrity and quality of the data, which is crucial for accurate analysis.
Data Transformation: Transform the data into a format suitable for analysis. This might include normalizing the data, encoding categorical variables, and creating new features if necessary.
python code
import pandas as pd
import numpy as np
# Load the data
data = pd.read_csv('water_flow_data.csv'
# Handle missing values
data = data.dropna()
# Feature scaling
data['scaled_water_level'] = (data['water_level'] - data['water_level'].mean()) / data['water_level'].std()
Visualization
Visualizing the data helps in understanding patterns, trends, and relationships between variables. It also aids in choosing the right non-linear model for the analysis.
Scatter Plots: Use scatter plots to visualize relationships between the dependent variable (e.g., water flow) and independent variables (e.g., time, temperature).
Histograms: Histograms help in understanding the distribution of variables, which is crucial for choosing appropriate transformations or models.
Line Plots: Line plots can show trends over time, which is particularly useful for time series data.
python code
import matplotlib.pyplot as plt
# Scatter plot
plt.scatter(data['time'], data['water_flow'])
plt.xlabel('Time'
plt.ylabel('Water Flow'
plt.title('Water Flow Over Time'
plt.show()
# Histogram
plt.hist(data['water_flow'], bins=2
plt.xlabel('Water Flow'
plt.ylabel('Frequency'
plt.title('Distribution of Water Flow'
plt.show()
Choosing a Non-Linear Model
Selecting the right non-linear model is critical for accurate predictions. Common non-linear models include polynomial regression, exponential models, and logarithmic models.
Polynomial Regression: Suitable for data with curvilinear relationships. The degree of the polynomial is chosen based on the data's complexity.
Exponential Models: Useful when data grows or decays exponentially.
Logarithmic Models: Appropriate when the rate of change decreases over time.
python code
from sklearn.preprocessing import PolynomialFeatures
# Polynomial regression
poly_features = PolynomialFeatures(degree=3)
X_poly = poly_features.fit_transform(data[['time']])
# Exponential regression example
def exponential_model(x, a, b):
return a * np.exp(b * x)
Model Fitting
Model fitting involves training the chosen non-linear model on the prepared data. This step typically includes selecting an appropriate loss function and optimization algorithm.
Training the Model: Fit the model to the training data and adjust parameters to minimize the error.
Cross-Validation: Use cross-validation to ensure the model generalizes well to unseen data.
python code
from sklearn.linear_model import LinearRegression
# Fitting polynomial regression model
model = LinearRegression()
model.fit(X_poly, data['water_flow'])
# Fitting exponential model using curve fitting
from scipy.optimize import curve_fit
params, _ = curve_fit(exponential_model, data['time'], data['water_flow'])
Model Evaluation
Evaluating the model is essential to assess its performance. Common metrics include Mean Squared Error (MSE), R-squared (R²), and visual inspections of residual plots.
Mean Squared Error (MSE): Measures the average squared difference between observed and predicted values.
R-squared (R²): Indicates the proportion of variance in the dependent variable that is predictable from the independent variables.
Residual Plots: Visualizing residuals helps in diagnosing issues like heteroscedasticity and non-linearity.
python code
from sklearn.metrics import mean_squared_error, r2_score
# Predictions
predictions = model.predict(X_poly)
# Evaluation
mse = mean_squared_error(data['water_flow'], predictions)
r2 = r2_score(data['water_flow'], predictions)
print(f'Mean Squared Error: {mse}'
print(f'R-squared: {r2}'
Assignment Example
To illustrate the application of non-linear regression in a practical scenario, consider an assignment example where you are tasked with predicting water flow based on various environmental factors.
Assignment Task:
Data Collection and Preparation: Gather water flow data and relevant environmental variables.
Visualization: Create scatter plots and histograms to understand the data.
Model Selection: Choose an appropriate non-linear model based on data visualization.
Model Fitting: Fit the model to the data.
Evaluation: Evaluate the model using MSE and R-squared metrics.
Report: Compile your findings, including visualizations, model parameters, and evaluation metrics.
python code
# Example Assignment Code
data = pd.read_csv('assignment_water_flow_data.csv'
data = data.dropna()
# Polynomial regression
poly_features = PolynomialFeatures(degree=2)
X_poly = poly_features.fit_transform(data[['time']])
model = LinearRegression()
model.fit(X_poly, data['water_flow'])
predictions = model.predict(X_poly)
mse = mean_squared_error(data['water_flow'], predictions)
r2 = r2_score(data['water_flow'], predictions)
print(f'Assignment MSE: {mse}'
print(f'Assignment R-squared: {r2}'
Conclusion
Non-linear regression is a powerful tool for water flow analysis, capable of capturing complex relationships that linear models cannot. By following a structured approach—from prerequisites and data preparation to model fitting and evaluation—you can effectively utilize non-linear regression for accurate predictions. Whether you're a student seeking online machine learning assignment help or a professional tackling real-world problems, mastering non-linear regression will significantly enhance your analytical capabilities.
By incorporating these steps into your workflow, you can unlock the full potential of non-linear regression, leading to more accurate and insightful water flow analyses.
Reference: https://www.programminghomewor....khelp.com/blog/non-l
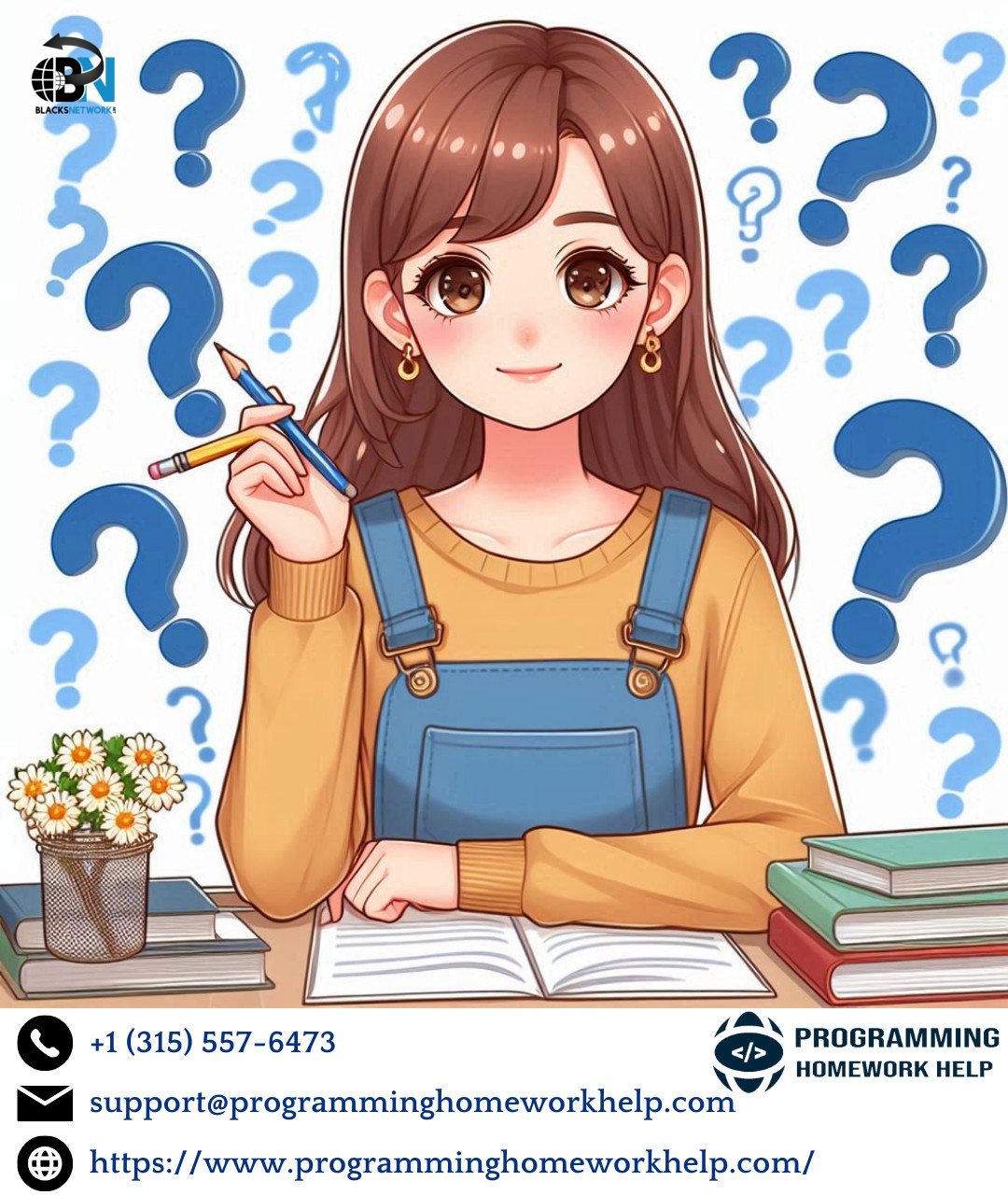