Mastering Programming Challenges with Expert Help: Python Assignment Solutions
In the rapidly evolving world of programming, students often face complex assignments that require a deep understanding of concepts and exceptional problem-solving skills. At https://www.programminghomewor....khelp.com/python-ass we specialize in providing high-quality assistance with programming assignments, particularly in Python. This blog explores how our expert Python assignment helpers tackle master-level programming questions, providing solutions that can serve as invaluable resources for students.
Complex Problem-Solving with Python
Python is renowned for its readability and versatility, making it a popular choice for various programming assignments. However, master-level Python assignments can be challenging, requiring advanced knowledge and problem-solving capabilities. Our Python assignment helpers are well-versed in addressing these challenges. Below are a couple of master-level questions we frequently encounter, along with detailed solutions.
Problem 1: Implementing a Custom Decorator for Memoization
Question: Implement a custom Python decorator to perform memoization for a recursive function that calculates the nth Fibonacci number. The decorator should cache the results of function calls to optimize performance and avoid redundant calculations.
Solution:
Memoization is a technique used to optimize recursive functions by caching previously computed results. This technique is particularly useful for functions like the Fibonacci sequence, where the same subproblems are solved multiple times. Here's how our expert Python assignment helper would approach this problem:
from functools import wraps
def memoize(func):
cache = {}
@wraps(func)
def memoized_func(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return memoized_func
@memoize
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
# Example usage
print(fibonacci(1) # Output: 55
In this solution, the memoize decorator is defined to cache the results of function calls. It uses a dictionary to store previously computed results, ensuring that each result is calculated only once. The fibonacci function, when decorated with @memoize, benefits from this optimization, drastically reducing the number of recursive calls.
Problem 2: Building a Custom Class with Advanced Iteration Capabilities
Question: Design a custom Python class that implements advanced iteration capabilities. The class should represent a collection of elements and provide a way to iterate over the elements in a custom order specified by a given function.
Solution:
Creating a custom iterable class involves implementing special methods like __iter__ and __next__. Our Python assignment helpers follow these steps to ensure that the custom class meets the required specifications:
class CustomCollection:
def __init__(self, elements):
self.elements = elements
def set_order(self, order_function):
self.order_function = order_function
def __iter__(self):
self.sorted_elements = sorted(self.elements, key=self.order_function)
self.index = 0
return self
def __next__(self):
if self.index < len(self.sorted_elements):
result = self.sorted_elements[self.index]
self.index += 1
return result
else:
raise StopIteration
# Example usage
collection = CustomCollection([5, 3, 9, 1])
collection.set_order(lambda x: -x) # Descending order
for item in collection:
print(item)
In this solution, the CustomCollection class allows setting a custom order for iteration through the set_order method. The __iter__ method sorts the elements based on the provided order function, and __next__ implements the iteration logic. This design allows for flexible and customizable iteration over the collection.
The Role of a Python Assignment Helper
At programminghomeworkhelp.com, our Python assignment helpers are dedicated to providing tailored solutions for complex programming problems. We understand that mastering advanced Python concepts can be daunting, especially when juggling multiple assignments. Our expert assistance ensures that students receive high-quality, comprehensive solutions that not only address the immediate problem but also enhance their understanding of key programming principles.
Why Choose Expert Help?
Deep Understanding: Our Python assignment helpers possess extensive knowledge and experience in advanced programming concepts, enabling them to tackle complex questions with precision.
Customized Solutions: We provide solutions tailored to the specific requirements of each assignment, ensuring relevance and clarity.
Learning Support: Beyond delivering answers, our services aim to educate and guide students, helping them grasp challenging concepts and improve their problem-solving skills.
Timely Delivery: We understand the importance of deadlines and ensure that all assignments are completed on time without compromising quality.
Conclusion
Complex programming assignments, particularly those involving advanced Python concepts, can be overwhelming for students. At programminghomeworkhelp.com, our expert Python assignment helpers are committed to providing the support and guidance needed to excel in these challenges. By leveraging our services, students can gain valuable insights, achieve higher grades, and develop a deeper understanding of programming principles.
If you're struggling with your Python assignments or need assistance with other programming tasks, don't hesitate to reach out. Our team is here to help you succeed in your academic journey.
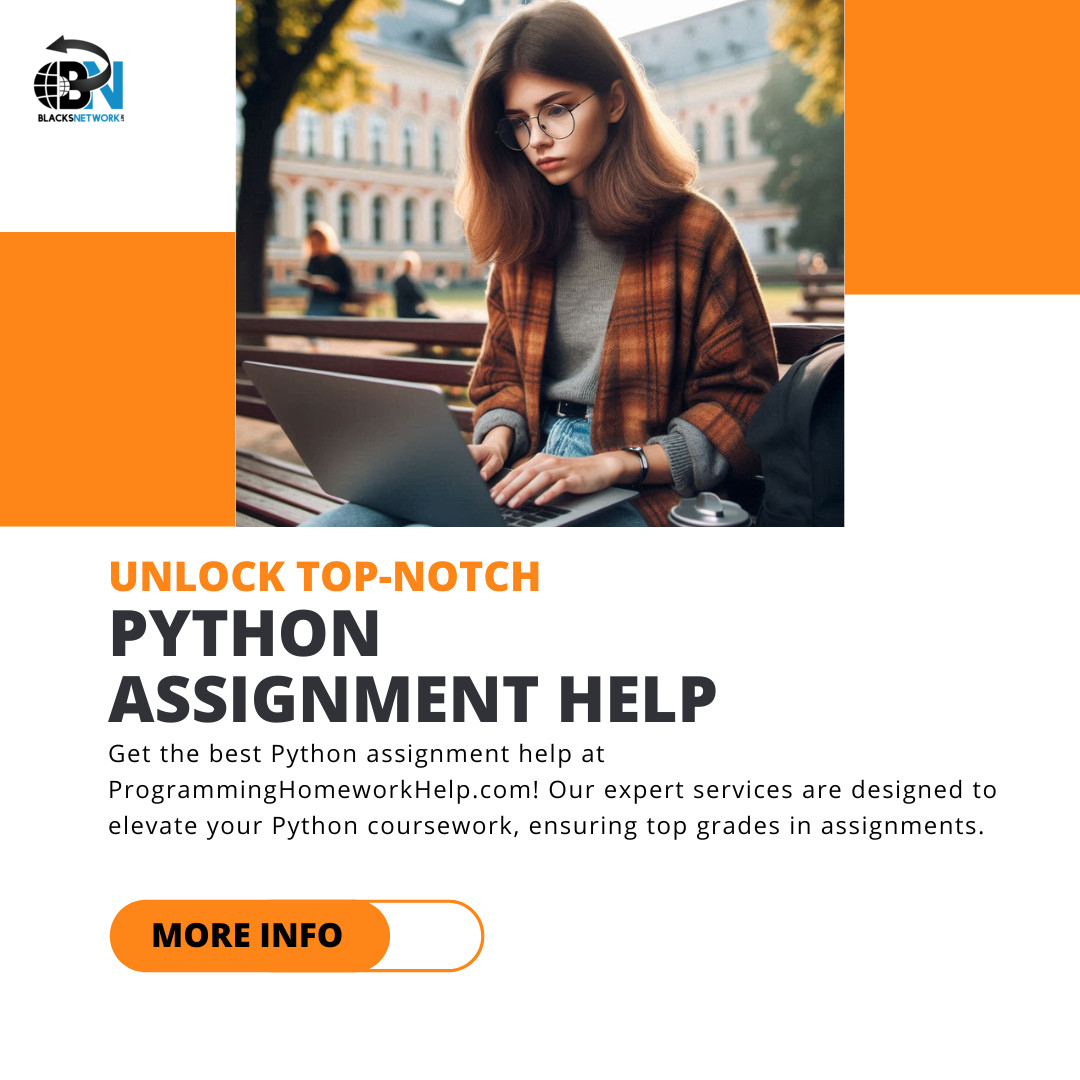